Compile API Models to Libraries
A Third-party compiler allows you to compile C++ code to a library
(.dll
in Windows or .so
in Linux).
EDEM does not support any specific compiler. However, Microsoft Visual Studio is commonly used and the Visual Studio Community version 2017 is used in this example along with Linux GCC.
The following example assumes you have already written the code to be compiled. The code used in this example is the Hertz-Mindlin model available on the Altair Community.
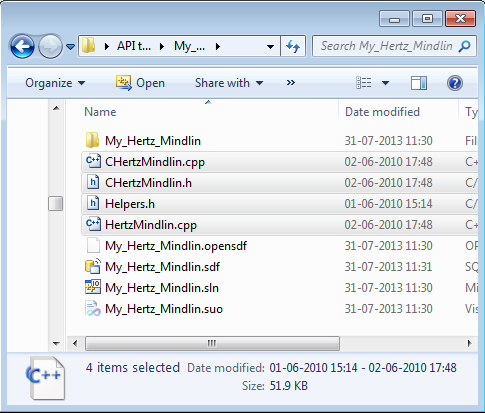
Windows
When installing Visual Studio, it is important to select to install the C++ tool set (Universal Windows Platform development and Desktop development with C++). Depending on the installation, these may be installed using default or additional options in the installer.
- Create a new Project.
- Open Visual C++ For Windows Desktop and go to .
- Select Win32 Console Application.
- Specify a project name and location and click OK.
- On the next window, go to Application Settings and select DLL in the Application Type dropdown list.
- Select Empty Project and then click
Finish.
- Copy your written
.cpp
and.h
files to the newly created project folder.Note: EDEM header files are also required before the model for compilation. The additional header files are available at the following location: . - Copy the following files from Core and Contact Models to your Project
folder:
IPluginContactModel.h
IPluginContactModelV3_3_0.h
PluginContactModelCore.h
ApiIds.h
ApiTypes.h
IApi.h
IApiManager_1_0.h
ICustomPropertyDataApi_1_0.h
ICustomPropertyManagerApi_1_0.h
PluginConstants.h
- Right-click Header files and Source
files to add the file to the Visual Studio Project.
- To compile the Contact Model, go to
- From Win32, select .
The Configuration Manager dialog box is displayed. - Select Release or Debug
and build the solution from .
A
.dll
file which can be loaded into EDEM is created.
. - From Win32, select .
Linux
g++
command, which is a common way of invoking a C++ compiler.
There are differences between the two commands, which we will not outline here, but
you should be able to use either when compiling your EDEM API plugin.- Use a compatible version of
g++
to compile plugins using EDEM’s API.Note: EDEM uses functionality from the C++11 standard, so you will require a compatible version ofg++
.g++ 4.8.1
was the first version to be fully compliant with the C++11 standard. If you have Red Hat/CentOS version 7 or later, you can use the default version ofg++
. You can check which version ofg++
you have by enteringg++ --version
in a terminal as follows: - To compile the Hertz-Mindlin example from the command line with an output
file of
HertzMindlinAPI.so
, use the following command:g++ -std=c++11 -O2 -shared -fPIC -I/home/<user>/<version number>/altair/src/Api/ContactModels/ -I/home/<user>/<version number>/altair/src/Api/Core/ -I/home/<user>/<version number>/altair/src/Misc/ CHertzMindlin.cpp HertzMindlin.cpp -o HertzMindlinAPI.so
- If you modify the installation path during the installation, you must update
the file locations to the appropriate directory.
<user>
must be updated to the appropriate account depending on the folder in which EDEM is installed:-std=c++11
informsg++
to expect functionality from the C++11 standard and use the appropriate libraries during compilation.-O2
specifies the use of the optimization method 2.Adding an optimization method flag allows
g++
to improve the performance of the resulting output file, though it potentially increases compilation time. Generally speaking, an EDEM API plugin will compile within seconds, so it is usually recommended to include this optimization option.-shared -fPIC
creates a shared object file as an output.The default output of
g++
is an executable program. Adding these flagsg++
produces a shared library instead.-I/<directory>
adds the directory to the included path.This allows you to use headers from a particular directory, without having to copy them to the directory location of your source code.
In addition to those mentioned above, when creating EDEM API plugins, you may also need to include the following:/home/<user>/<version number>/altair/src/Api/Factories
/home/<user>/<version number>/altair/src/Api/ParticleBodyForce
- Alternatively, you can copy the relevant header files to your
working directory if you do not want to use this flag to include any
directories.
-o
specifies the output file name. This is not mandatory, but is required if you want the created shared library to have a particular name. You can rename the file after compilation if you do not use this flag. This is a single command, and only spaces but not new lines between the arguments are allowed. The arguments are displayed in new lines for easier readability. - Enter the following command (script):
g++ -std=c++11 -02 -fPIC -I/home/<user>/2023/altair/src/API/ContactModels/ -I/home/<user>/2023/altair/src/API/Core/ I/home/<user>/2023/altair/src/API/Misc/ CHertzMindlin.cpp HertzMindlin.cpp -o HertMindlinApi.so
Note: It is recommended to specify the compile flags appropriate to their model and requirements, though the above flags are commonly used and found to work when compiling EDEM API plugins. For more information about flags for g++, see https://g++.gnu.org/ or referg++’
s man entry (man g++
).
Create a Compilation Script
- Use a text editor such as Emacs, Vim or Gedit, and create a new text file
similar to the previous command (edited for your particular filenames and
included directories) and save it with a file name of your choice such
as
compileScript
, in the same folder as your source code.
In this case, Vim is used, which should be available to all Linux distributions, though the text editors available to you might mean it is easier to use something else.
- Navigate to the directory where you have saved your script using the
cd
command, and then make the script an executable using the commandchmod 755 compileScript
.
When you list the contents of the directory using the
ls
command, thecompileScript
has executable permissions (which now shows in green). - Run the compile script using
./compileScript
and your.so
file is created.
- When compiling another model, you must change the included directories and file names in this script, or modify the script to accept arguments instead.