stft
Short-Time Fourier Transform.
Syntax
st = stft(x)
st = stft(x,fs)
st = stft(x,fs,window)
st = stft(x,fs,window,overlap)
st = stft(x,fs,window,overlap,nfft)
st = stft(x,fs,window,overlap,nfft)
st = stft(x,fs,window,overlap,nfft,range)
[st,t,f] = stft(...)
Inputs
- x
- The signal to be transformed into the frequency domain.
- fs
- The sampling frequency.
- window
- The window size, or the window column vector.
- overlap
- The number of overlapping points in adjacent windows.
- nfft
- The size of the fft.
- range
- The spectrum type. The options are as follows:
- 'onesided' (default)
- 'onesided_dB'
- 'twosided'
Outputs
- st
- The short-time fourier transform output, with segments stored by column.
- t
- The vector of times corresponding to the start of each column of st.
- f
- The vector of frequencies corresponding to each row of st.
Example
stft of a linear chirp signal.
f0 = 0;
f1 = 5;
T = 10;
c = (f1 - f0) / T;
t = [0:0.1:T-0.1];
x = sin(2 * pi * ((c/2)*t.^2 + f0*t));
stft(x, 10, 20, 14, 32, 'onesided');
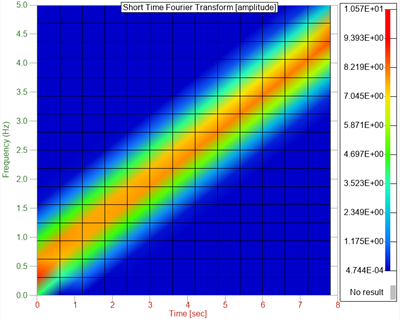
Figure 1. stft figure 1
Comments
Default values are assigned to arguments with a [] input.
The function produces unnormalized spectral values with no compensation for the window bias. It may be useful to normalize st by sum(win), where win is the window vector.
The 'onesided' and 'onesided_dB'outputs have a length of nfft/2+1 if nfft is even, or (nfft+1)/2 if nfft is odd.
It is often recommended to remove the trend line prior to calling stft. The function does not automatically call detrend.