Entity Selection#
Some examples require external input files. Before you start, please follow the link in the Example Scripts section to download the zip file with model and result files.
Example 01 - Part lists with Collections and evalTcl()#
Part lists with Collections and evalTcl() postquery#
1import hw
2import hw.hv as hv
3import os
4
5scriptDir = os.path.abspath(os.path.dirname(__file__))
6modelFile = os.path.join(scriptDir, "aerobox", "aerobox.fem")
7resultFile = os.path.join(scriptDir, "aerobox", "aerobox-LC1-2.op2")
8
9ses = hw.Session()
10ses.new()
11page = ses.get(hw.Page)
12win = ses.get(hw.Window)
13win.type = "animation"
14win.addModelAndResult(modelFile, result=resultFile)
15animTool = hw.AnimationTool()
16animTool.currentFrame = 1
17hw.evalHWC("view orientation iso")
18
19print()
20print("Part lists by evalTcl() with postquery package")
21hw.evalTcl("package require postquery")
22partList = [int(x) for x in hw.evalTcl("::hwp::getComponentList").split()]
23print("Part ID List = ", partList)
24partNameList = hw.evalTcl("::hwp::getComponentList -byname 1").split()
25print("Part Name List = ", partNameList)
26
27print()
28print("Part lists by Collections")
29partCol = hv.Collection(hv.Part)
30partList = [p.id for p in partCol.getEntities()]
31print("Part ID List = ", partList)
32partNameList = [p.name for p in partCol.getEntities()]
33print("Part Name List = ", partNameList)
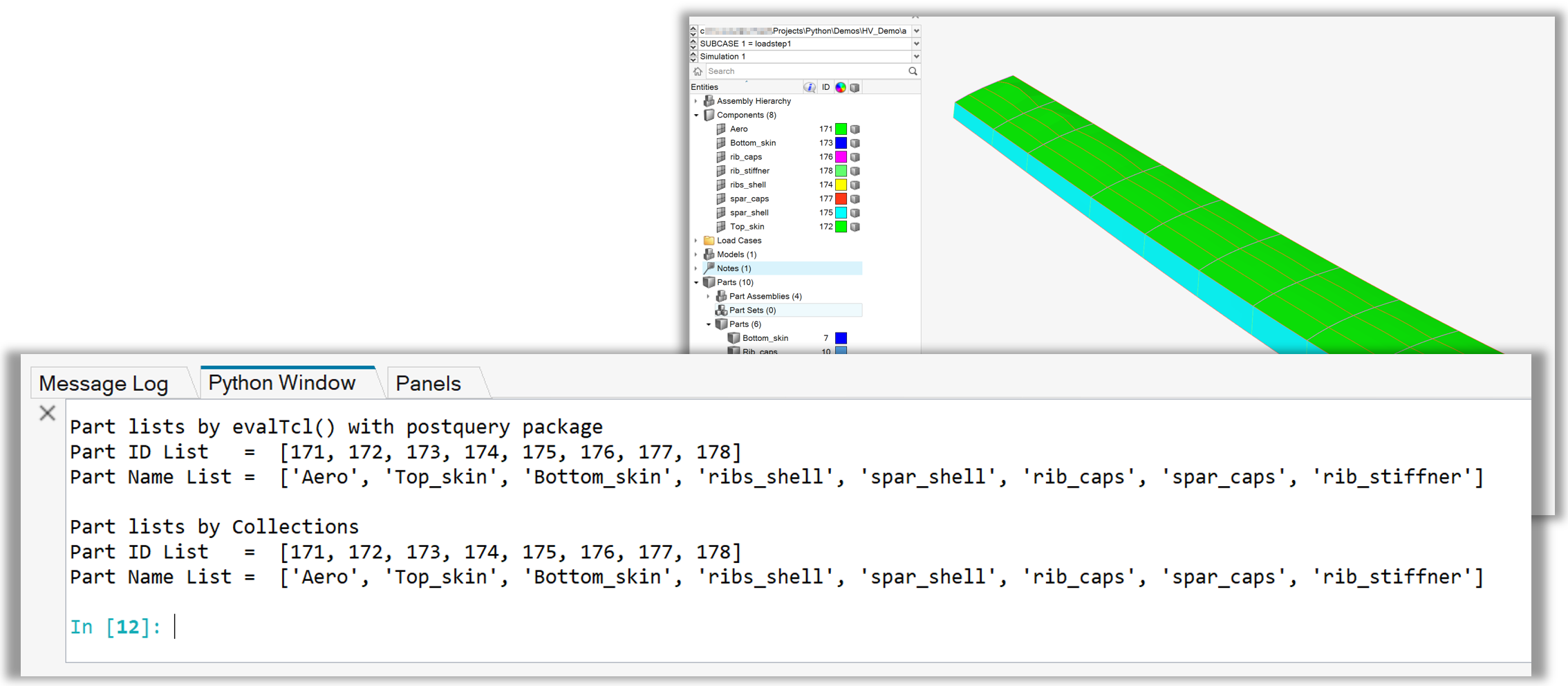
Figure 1. Output of ‘Part lists with Collections and evalTcl() postquery’
Example 02 - Interactive Selection with Default Settings#
Interactively querying Parts using custom context with default settings#
1import hw
2import hw.hv as hv
3import os
4
5ALTAIR_HOME = os.path.abspath(os.environ['ALTAIR_HOME'])
6modelFile = os.path.join(ALTAIR_HOME,'demos','mv_hv_hg','animation','h3d','NEON_FRONT.h3d')
7
8ses = hw.Session()
9ses.new()
10win=ses.get(hw.Window)
11# Set Window to Animation
12win.type = 'animation'
13# Add Model
14win.addModelAndResult(modelFile)
15
16# Interactive Selection with default settings
17inSel = hv.InteractiveSelection()
18pCol=inSel.select()
19print('')
20print('Interactive Selection with Defaults')
21print('-----------------------------------')
22print('Selection type = {}'.format(inSel.type))
23print('Selection mode = {}'.format(inSel.mode))
24print('Selection modal = {}'.format(inSel.modal))
25print('Selection message = {}'.format(inSel.message))
26print('Collection size = {}'.format(pCol.getSize()))
27print('{} selected'.format(inSel.type))
28
29for p in pCol.getEntities()[:10]:
30 print(' Part id = {} name = {}'.format(p.id,p.name))
31print(' ...')
32win.draw()
Figure 2. Custom Context at interactive Part selection using default settings
Example 03 - Interactive sorted Node List Selection#
Interactive modal selection of a sorted note list with status bar message#
1import hw
2import hw.hv as hv
3import os
4import numpy as np
5
6ALTAIR_HOME = os.path.abspath(os.environ['ALTAIR_HOME'])
7modelFile = os.path.join(ALTAIR_HOME,'demos','mv_hv_hg','animation','h3d','NEON_FRONT.h3d')
8
9ses = hw.Session()
10ses.new()
11win=ses.get(hw.Window)
12# Set Window to Animation
13win.type = 'animation'
14# Add Model
15win.addModelAndResult(modelFile)
16
17inSel = hv.InteractiveSelection(type='Node',
18 modal=True,
19 mode='list',
20 message='Select Nodes into sorted List'
21 )
22nList=inSel.select()
23print('Selection type = {}'.format(inSel.type))
24print('Selection mode = {}'.format(inSel.mode))
25print('Selection modal = {}'.format(inSel.modal))
26print('Selection message = {}'.format(inSel.message))
27print('List length = {}'.format(len(nList)))
28pathLength = 0
29ns = nList[0]
30print(' Start node = {}'.format(ns.id))
31for n in nList:
32 pathLength += np.linalg.norm(np.array(n.coords) - np.array(ns.coords))
33 print(' Path length to node {} = {}'.format(n.id,pathLength))
34 ns = n
Figure 3. Custom context of interactive sorted Node list selection