Examples#
Some examples require external input files. Before you start, please follow the link in the Example Scripts section to download the zip file with model and result files.
Example 01 - Using hm and hm.entities module#
Model setup using HyperMesh Python API#
1# ---Imports-----------
2import hm
3import hm.entities as ent
4import os
5
6
7def main():
8 # Grabbing a model instance by name
9 session = hm.Session()
10 model = hm.Model(session.get_all_models()[0])
11
12 # Reading HyperMesh model
13 script_dir = os.path.dirname(os.path.abspath(__file__))
14 model.readfile(os.path.join(script_dir,"pedal.hm"),0)
15
16 # Material Creation
17 mat1 = ent.Material(model)
18 mat1.name = "Steel"
19 mat1.cardimage = "MAT1"
20 mat1.E = 21e04
21 mat1.Nu = 0.3
22 mat1.Rho = 7850
23
24 # Property Creation and Material Assignment to Property
25 prop1 = ent.Property(model)
26 prop1.name = "Prop"
27 prop1.cardimage = "PSOLID"
28 prop1.materialid = mat1
29
30 # Assign Properties to Component with id=1
31 compID = 1
32 comp1 = ent.Component(model, compID)
33 comp1.property = prop1
34
35 # Create Tetramesh
36 node_col = hm.Collection(model, ent.Node, populate=False)
37 str_array1 = hm.hwStringList(
38 [
39 "pars: upd_shell fix_comp_bdr post_cln elem_order = 2 delaunay el2comp=3 fill_void=1 tet_clps='0.100000,0.300000, 0.500000, 1.000000, 0.380000, 0.100000'",
40 "tet: 35 1.3 -1 0.014 0.8 0 0 1",
41 "2d: 1 0 4 0.01 0.001 30 1",
42 ]
43 )
44 solids_col = hm.Collection(model, ent.Solid)
45 model.tetmesh(
46 collection1=solids_col,
47 mode1=1,
48 collection2=node_col,
49 mode2=5,
50 string_array=str_array1,
51 )
52
53 # Apply constraints to specific nodes
54 indNode = ent.Node(model, 1462) # Independent Node for rigidlink
55 nodelist = (
56 list(range(1420, indNode.id))
57 + list(range(indNode.id + 1, 1642))
58 + list(range(74051, 74064))
59 + list(range(74214, 74300))
60 + list(range(74356, 74369))
61 + list(range(74980, 75066))
62 )
63
64 # Dependent Node for rigidlink
65 filtNode = hm.FilterByEnumeration(ent.Node, nodelist)
66 depnodes = hm.Collection(model, filtNode)
67 model.rigidlink(
68 independent=indNode, collection=depnodes, dofs=123456
69 ) # rigid link creation
70 indNode_col = hm.Collection(model, ent.Node, f"id={indNode.id}")
71 model.loadcreateonentity_curve(
72 collection=indNode_col,
73 config=3,
74 type=1,
75 comp1=0,
76 comp2=0,
77 comp3=0,
78 comp4=0,
79 comp5=0,
80 comp6=0,
81 x_loc=0,
82 y_loc=0,
83 z_loc=0,
84 curve_id=0,
85 x_scale=0,
86 )
87
88 # Creating a Load collection with the name "Pressure" and we apply it on selected elements
89 loadcol2 = ent.Loadcol(model)
90 loadcol2.name = "Pressure"
91
92 elemes = hm.CollectionByInteractiveSelection(model, ent.Element)
93 filt = hm.FilterByCollection(ent.Node, ent.Element, [""])
94 nele = hm.Collection(model, filt, elemes)
95
96 model.pressuresonentity_curve(
97 collection=elemes,
98 facenodes=nele,
99 x_comp=0,
100 y_comp=0,
101 z_comp=-1,
102 magnitude=1,
103 breakangle=30,
104 onface=1,
105 xlocation=0,
106 ylocation=0,
107 zlocation=0,
108 curve_id=0,
109 x_scale=0,
110 )
111
112 # Setting up the loadstep
113 loadStep1 = ent.Loadstep(model)
114 loadStep1.OS_TYPE = 1 # Linear Static
115 loadStep1.OS_SPCID = model.get(hm.Entity(ent.Loadcol, 1))
116 loadStep1.OS_LOADID = loadcol2
117
118 # Two options for our geometry. Translation of elements or rotation
119 opt = int(
120 input("Enter Option: 1 for Translate Elements, 2 for Component Rotation: ")
121 )
122 if opt == 1:
123 # Translate elements
124 tranElem = hm.CollectionByInteractiveSelection(model, ent.Element)
125 model.translatemark(collection=tranElem, vector=[0.0, 1.0, 0.0], distance=0.5)
126 elif opt == 2:
127 # Rotate component
128 comp1 = hm.Collection(model, ent.Component, "id=1")
129 RotAx = [0.0, 0.0, 1.0]
130 PlaneOr = [0.0, 0.0, 0.0]
131 model.rotatemark(
132 collection=comp1, plane_normal=RotAx, plane_normal_base=PlaneOr, angle=30
133 )
134
135
136if __name__ == "__main__":
137 main()
Example 02 - Querying the properties of a model#
Query the property entities and for selected elements query their property, material, and jacobian#
1import hm
2import hm.entities as ent
3import prettytable as pt
4
5model = hm.Model()
6
7# Create a collection of all properties in the model
8prop_data = list()
9props = hm.Collection(model, ent.Property)
10
11# Printing a table of properties and their respective material if exist
12for p in props:
13 prop_name = p.name
14 prop_id = p.id
15 prop_cardimage = p.cardimage
16 if prop_cardimage == "PSHELL":
17 prop_thickness = p.PSHELL_T
18 else:
19 prop_thickness = "-"
20 if p.materialid:
21 mat = p.materialid
22 mat_name = mat.name
23 mat_id = mat.id
24 mat_cardimage = mat.cardimage
25 else:
26 mat_name = "-"
27 mat_id = "-"
28 mat_cardimage = "-"
29 prop_data.append(
30 [
31 prop_name,
32 prop_id,
33 prop_cardimage,
34 prop_thickness,
35 mat_name,
36 mat_id,
37 mat_cardimage,
38 ]
39 )
40
41tab = pt.PrettyTable(
42 [
43 "Prop Name",
44 "Prop ID",
45 "Prop Cardimage",
46 "Prop Thickness",
47 "Mat Name",
48 "Mat ID",
49 "Mat Cardimage",
50 ]
51)
52
53tab.add_rows(prop_data)
54print(tab)
55
56# Create a collection of elements by interactive selection
57elems = hm.CollectionByInteractiveSelection(model, ent.Element)
58
59# Create a table of elements along with some attributes (id, configuration number, property_name, material_name, No of nodes and jacobian)
60elem_data = list()
61
62for e in elems:
63 el_id = e.id
64 el_conf = e.config
65 el_ncount = e.nodecount
66 if e.propertyid:
67 el_prop_name = e.propertyid.name
68 else:
69 el_prop_name = "-"
70 if e.materialid:
71 el_mat_name = e.materialid.name
72 else:
73 el_mat_name = "-"
74 if e.jacobian:
75 el_jac = e.jacobian
76 else:
77 el_jac = "-"
78 elem_data.append(
79 [
80 el_id,
81 el_conf,
82 el_ncount,
83 el_prop_name,
84 el_mat_name,
85 el_jac
86 ]
87)
88
89tab2 = pt.PrettyTable(
90 [
91 "Elem ID",
92 "Elem Config",
93 "Elem NodeCount",
94 "Elem Prop",
95 "Elem Mat",
96 "Jacobian",
97 ]
98)
99tab2.add_rows(elem_data)
100print(tab2)
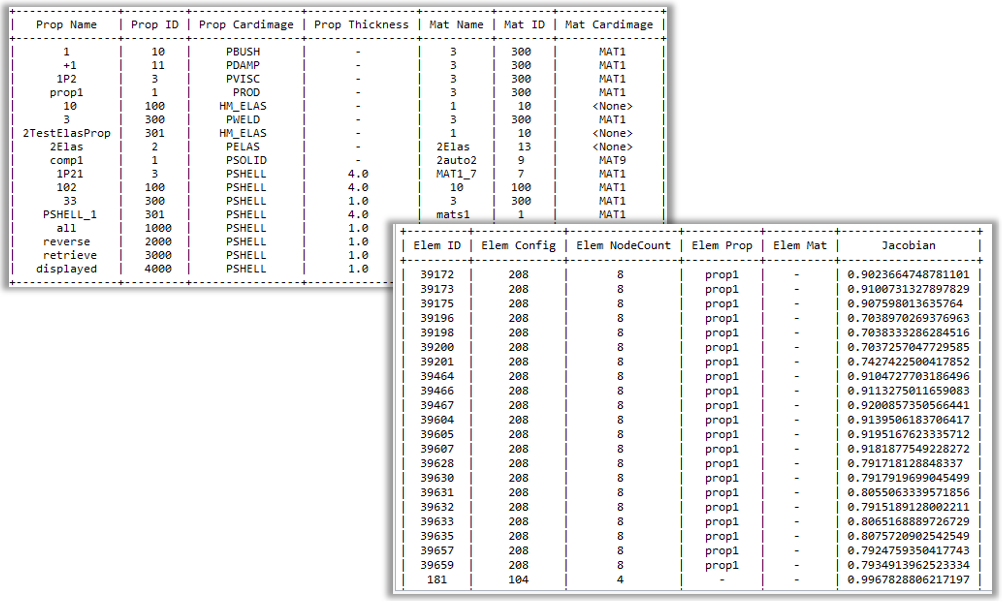
Figure 1. Querying elements’ and properties’ data
Example 03 - Creating a node at CoG of every component#
For each component, assign a property and a material, and create node at the center of gravity (CoG).#
1import hm
2import hm.entities as ent
3
4model = hm.Model()
5
6# Importing the model in HyperMesh
7datadir = rf"{hm.altair_home}\demos\hm"
8model.hm_answernext("yes")
9model.readfile(
10 filename=os.path.join(datadir, "bumper.hm"),
11 load_cad_geometry_as_graphics=0,
12)
13
14# Create a material with name 'Al' and properties of Aluminum
15mat1 = ent.Material(model)
16mat1.name = "Al"
17mat1.cardimage = "MAT1"
18mat1.E = 7e04
19mat1.Nu = 0.3
20mat1.Rho = 2700
21
22# Create a property with the name 'central_prop' and assign 'Al' material to it
23prop1 = ent.Property(model)
24prop1.name = "central_prop"
25prop1.cardimage = "PSHELL"
26prop1.materialid = mat1
27prop1.PSHELL_T = 1.0
28
29# Assign the property to all components, compute for each component the center of gravity (CoG) and a create a node to cog
30comp_col = hm.Collection(
31 model, ent.Component
32)
33
34# Create a collection of all components
35for comp in comp_col:
36 comp.propertyid = prop1
37 ccol = hm.Collection(model, ent.Component, [comp.id])
38 status, result = model.hm_getcog(ccol)
39 nodeNew = ent.Node(model) # Create a new node
40 nodeNew.localcoordinates = result.coord # Place the node at CoG
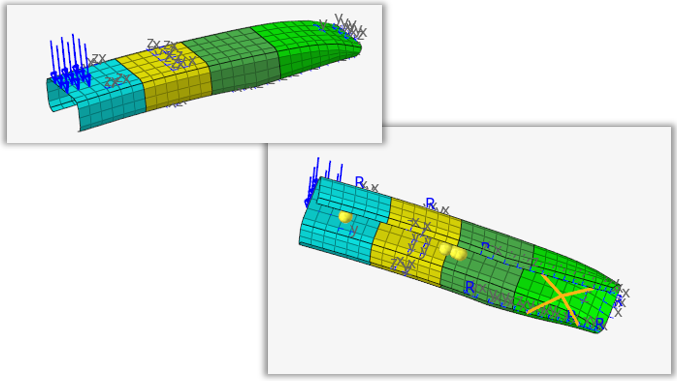
Figure 2. Creating nodes at components COGs
Example 04 - Creating a new component per solid (includes “performance” API)#
Create a new component for each solid while boosting the performance using the corresponding setoption options.#
1import hm
2import hm.entities as ent
3
4
5def main():
6
7 model = hm.Model()
8
9 solidcol = hm.Collection(model, ent.Solid)
10
11 # Enable performance boost
12 performance(model, True)
13
14 # Create a component for each solid
15 for solid in solidcol:
16 comp = ent.Component(model)
17 comp.name = f"solid_{solid.id}"
18 # Organize (move) the solid to the new component
19 filterBen = hm.FilterByEnumeration(ent.Solid, list([solid.id]))
20 solid_col = hm.Collection(model, filterBen)
21 model.movemark(collection=solid_col, name=f"solid_{solid.id}")
22
23 # Disable performance boost
24 performance(model, False)
25
26
27def performance(model, switch):
28 if switch == True:
29 hm.setoption(
30 block_redraw=1,
31 command_file_state=0,
32 entity_highlighting=0,
33 )
34 model.hm_blockbrowserupdate(mode=1)
35 else:
36 hm.setoption(
37 block_redraw=0,
38 command_file_state=1,
39 entity_highlighting=1,
40 )
41 model.hm_blockbrowserupdate(mode=0)
42
43
44if __name__ == "__main__":
45 main()