Examples#
Example 01 - Curves from Expression#
Create two curves from math expressions#
1from hw import *
2from hw.hg import *
3import os
4
5ses = Session()
6ses.new()
7win = ses.get(Window)
8win.type = "xy"
9
10exprXlist = ["0:1:0.0001", "c1.x"]
11exprYlist = ["sin(x*2*PI)", "c1.y+0.2*sin(60*x*2*PI)"]
12labelList = ["Basic Oscillation 1 HZ", "Overtone 60 HZ"]
13
14for exprX, exprY, label in zip(exprXlist, exprYlist, labelList):
15 cu = CurveXY(
16 xSource="math",
17 xExpression=exprX,
18 ySource="math",
19 yExpression=exprY,
20 label=label,
21 )
22
23win.update()
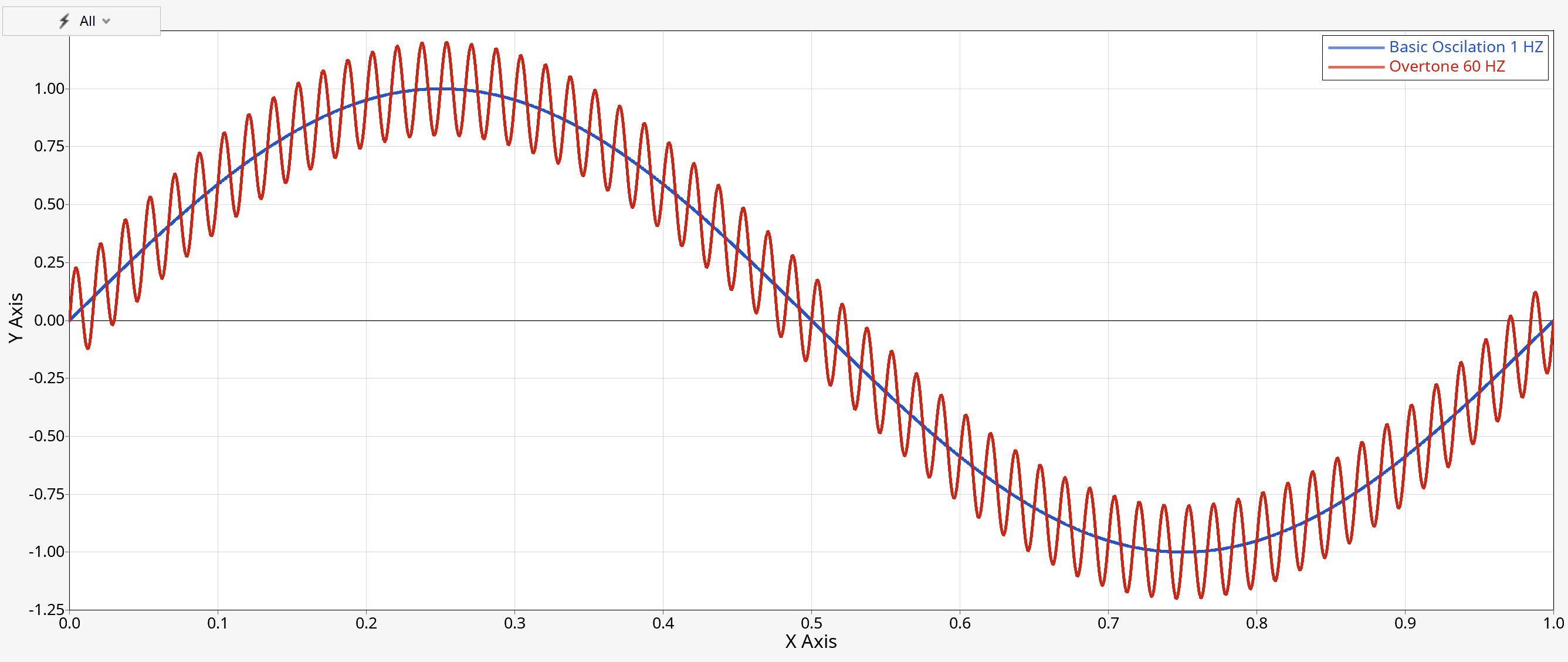
Figure 6. Output of ‘Create two curves from math expressions’
Example 02 - Curves from File#
Load two curves from file, define color and label#
1from hw import *
2from hw.hg import *
3import os
4
5ALTAIR_HOME = os.path.abspath(os.environ["ALTAIR_HOME"])
6plotFile = os.path.join(
7 ALTAIR_HOME, "demos", "mv_hv_hg", "plotting", "madymo", "ANGACC"
8)
9
10ses = Session()
11ses.new()
12win = ses.get(Window)
13win.type = "xy"
14
15requestList = ["50th% Hybrid3 - LOWER TORSO", "50th% Hybrid3 - UPPER TORSO"]
16colorList = [(255, 0, 0), (0, 0, 255)]
17
18for color, request in zip(colorList, requestList):
19 cu = CurveXY(
20 xFile=plotFile,
21 xSource="file",
22 xDataType="Time",
23 xRequest="Time",
24 xComponent="Time",
25 yFile=plotFile,
26 ySource="file",
27 yDataType="Angular Acceleration",
28 yRequest=request,
29 yComponent="Res. ang. acc.",
30 lineColor=color,
31 label="{y.HWRequest} - {y.HWComponent}",
32 )
33
34win.update()
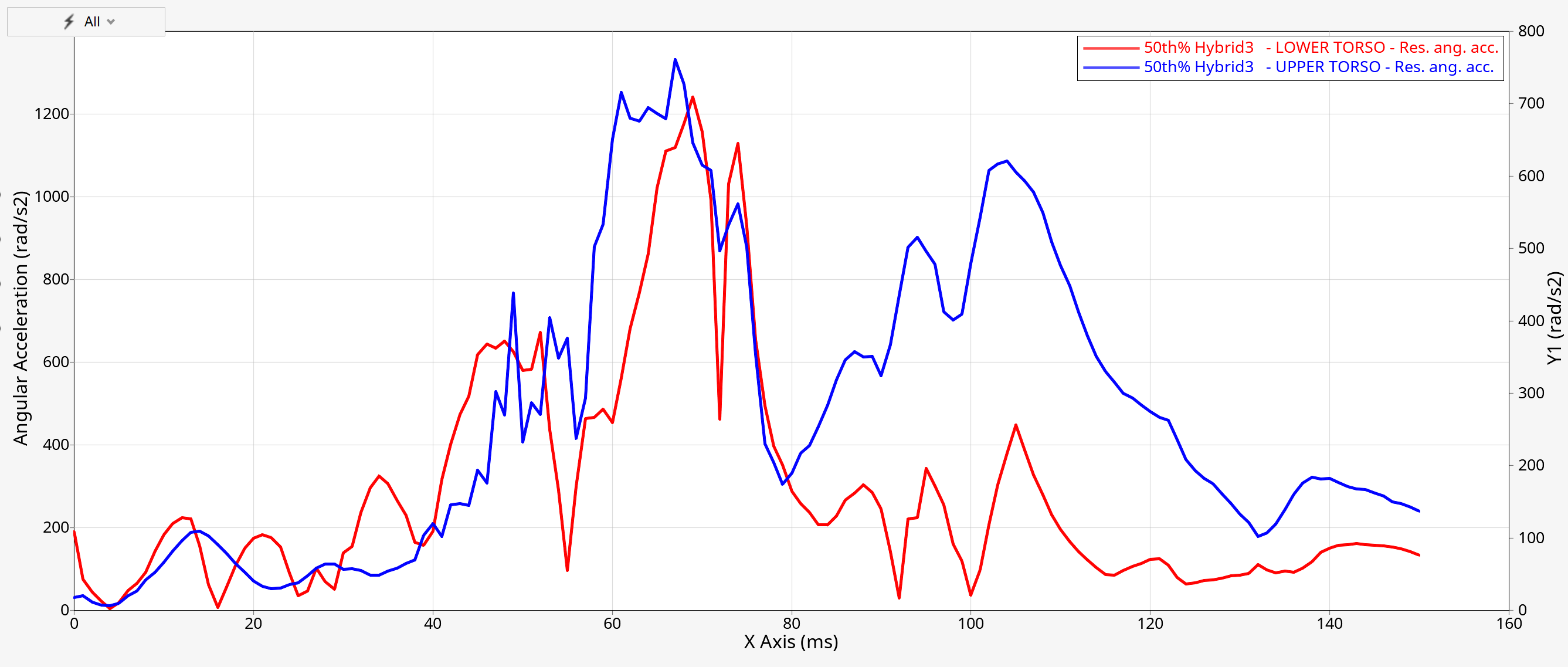
Figure 7. Output of ‘Load two curves from file, define color and label’
Example 03 - Curves from Files, Datums, Notes, Legends and PNG, XYDATA Export#
Load two curves, shade the area between, add notes and datums and export PNG#
1from hw import *
2from hw.hg import *
3import os
4
5ALTAIR_HOME = os.path.abspath(os.environ["ALTAIR_HOME"])
6plotFile = os.path.join(
7 ALTAIR_HOME, "demos", "mv_hv_hg", "plotting", "madymo", "ANGACC"
8)
9jpgFile = os.path.join(
10 os.path.abspath(os.path.dirname(__file__)), "HG_Image_Export.png"
11)
12xyDataFile = os.path.join(
13 os.path.abspath(os.path.dirname(__file__)), "HG_Curve_Export.xydata"
14)
15
16# New session
17ses = Session()
18ses.new()
19win = ses.get(Window)
20win.type = "plot"
21
22# Define image and curve export tools
23captureImage = CaptureImageTool(
24 file=jpgFile,
25 type="png",
26 width=2000,
27 height=1000,
28)
29exportCurves = ExportCurves(file=xyDataFile, format="xyData", range="all")
30
31# Layout and channel settings
32colorList = [(255, 0, 0), (0, 0, 255)]
33requestList = ["50th% Hybrid3 - LOWER TORSO", "50th% Hybrid3 - UPPER TORSO"]
34autoPosList = ["right", "left"]
35
36# Loop over curves, notes and datums
37for color, request, autoPos in zip(colorList, requestList, autoPosList):
38
39 # Add curves
40 cu = CurveXY(
41 xFile=plotFile,
42 xSource="file",
43 xDataType="Time",
44 xRequest="Time",
45 xComponent="Time",
46 yFile=plotFile,
47 ySource="file",
48 yDataType="Angular Acceleration",
49 yRequest=request,
50 yComponent="Res. ang. acc.",
51 lineColor=color,
52 label="{y.HWRequest} - {y.HWComponent}",
53 )
54
55 # Add curve math
56 cu.mathAdd("SAE", padding=1, direction=1, filterClass=15)
57 cu.mathAdd("trim", start=20, end=130)
58
59 cuId = str(cu.id)
60
61 # Add note attached to curve math
62 no = Note(
63 attachTo="curve",
64 attachmentCurve=cu.id,
65 attachmentPoint="indexofmax(c" + cuId + ".y)",
66 text="Max = {y} at {x}",
67 autoPosition=True,
68 autoPositionStrategy=autoPos,
69 curveColor=True,
70 )
71
72 # Add vertical datum
73 dv = DatumVertical(
74 position="c" + cuId + ".x[indexofmax(c" + cuId + ".y)]",
75 label="Max " + cu.yRequest,
76 labelLocation="upperCenter",
77 lineColor=color,
78 )
79
80# Modify legend
81legend = ses.get(Legend)
82legFont = Font(size=14)
83legend.placement = "insideRightBottomCorner"
84legend.font = legFont
85
86# Shade area between 2 curves
87cu.setAttributes(
88 shadeArea=True,
89 shadeStyle="betweenCurves",
90 shadeSecondCurve=1,
91 shadeColor="Blue",
92 shadeAlpha=0.2,
93)
94
95win.update()
96
97# Export image and modified curve values in XYDATA format
98captureImage.capture()
99exportCurves.export()
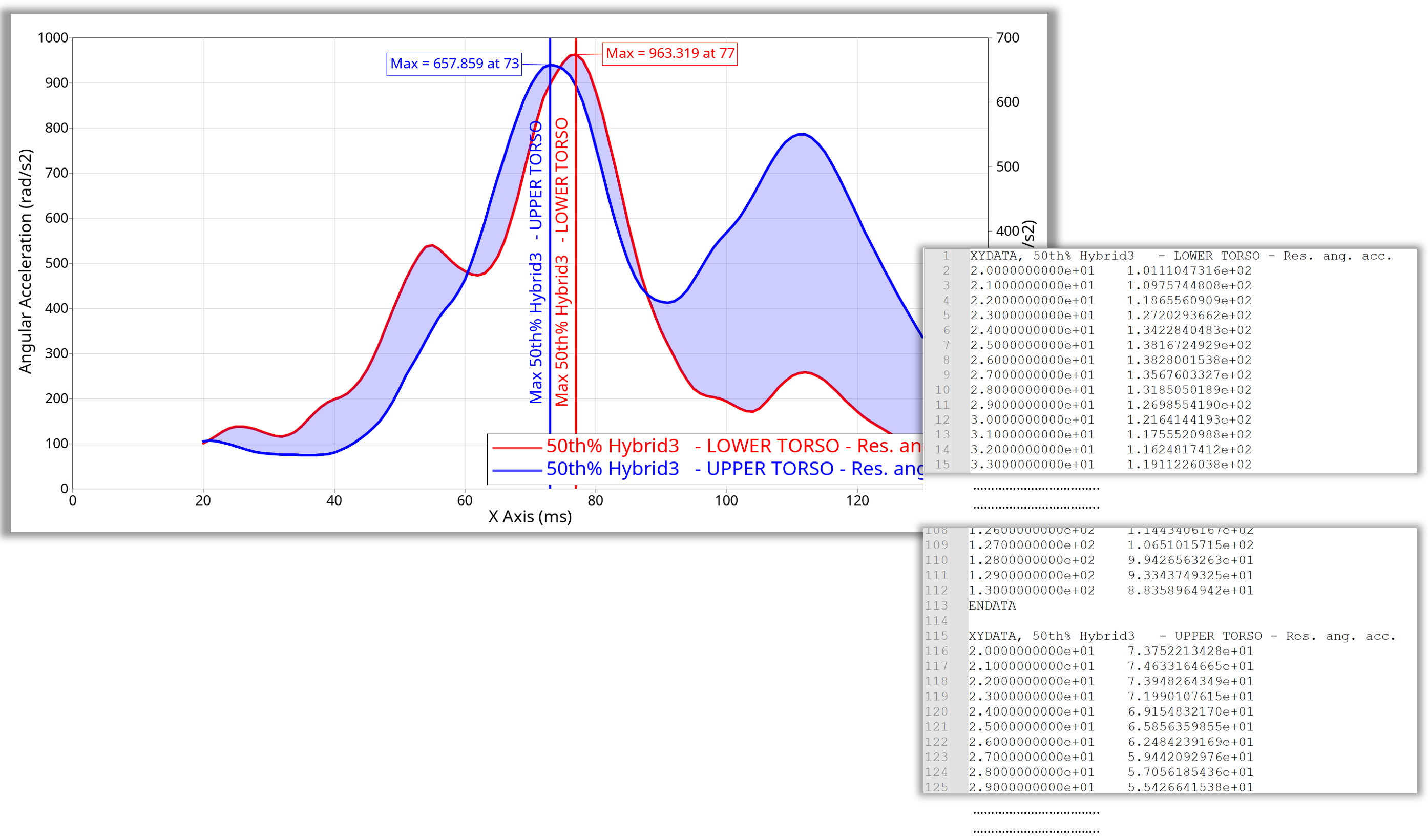
Figure 8. Exported Image of script ‘Curves from Files, Datums, Notes, Legends and PNG, XYDATA Export’
Example 04 - evalHWC() for Curve Import and Math Operations#
evalHWC() - Curve Import and Math Operations#
1from hw import *
2from hw.hg import *
3import os
4
5src_path = os.path.dirname(os.path.abspath(__file__))
6plotFile = os.path.join(src_path, "ANGACC")
7
8# New session
9evalHWC("delete session")
10# Set Client Type to HyperGraph
11evalHWC('hwd window type="HyperGraph 2D"')
12# Load curves with build plots module
13evalHWC("xy option layout destination=oneplotperrequest")
14evalHWC("xy option layout layout=1")
15evalHWC(
16 "xy load file="
17 + plotFile
18 + ' ydatatype= "Angular Acceleration" \
19 yrequest= "50th% Hybrid3 - LOWER TORSO" , "50th% Hybrid3 - UPPER TORSO" ycomponent= all'
20)
21evalHWC(
22 'xy curve stackadd range="w:all i:all" operation=trim start=20 end=130 interpolate=false'
23)
24# Add curve math for all curves in current plot
25evalHWC(
26 'xy curve stackadd range="w:all i:all" operation=SAE padding=1 samples=10 direction=3 filterClass=60'
27)
28evalHWC(
29 'xy curve stackadd range="w:all i:all" operation=resample type="Resample Fixed Points" fixnum=100.0'
30)
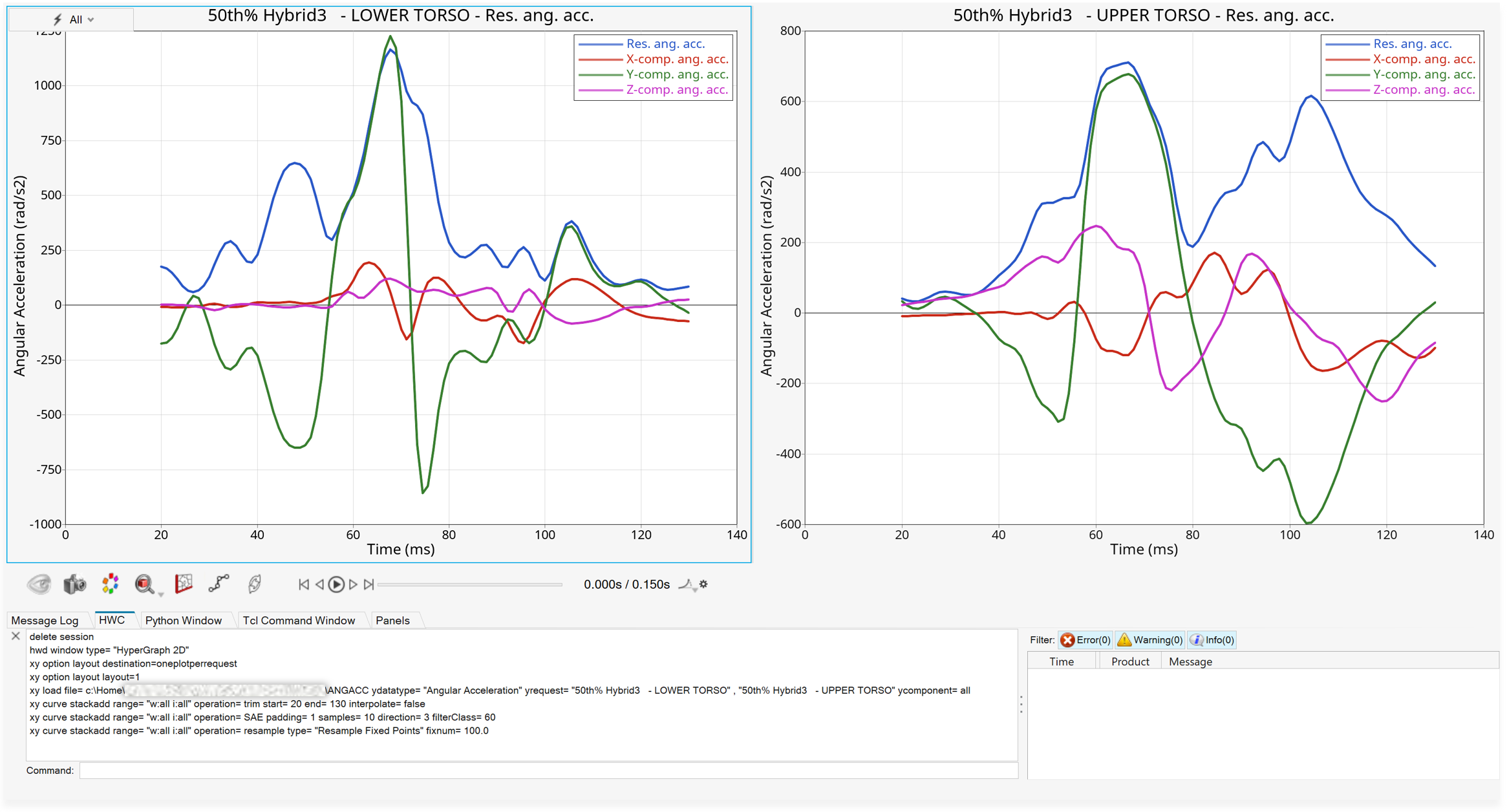
Figure 9. Output of ‘evalHWC() for Curve Import and Math Operations’
Example 05 - Create Multiple Plot Layout#
Create multiple pages from channel info stored in dictionary#
1from hw import *
2from hw.hg import *
3import os
4
5ALTAIR_HOME = os.path.abspath(os.environ["ALTAIR_HOME"])
6plotFile = os.path.join(
7 ALTAIR_HOME, "demos", "mv_hv_hg", "plotting", "madymo", "ANGACC"
8)
9
10# New Session
11ses = Session()
12ses.new()
13
14# Dictionary with curve channel names
15plotDict = {
16 "yRequests": [
17 "50th% Hybrid3 - LOWER TORSO",
18 "50th% Hybrid3 - UPPER TORSO",
19 "50th% Hybrid3 - UPPER LEG RIGHT",
20 "50th% Hybrid3 - UPPER LEG LEFT",
21 ],
22 "yComponents": [
23 "Res. ang. acc.",
24 "X-comp. ang. acc.",
25 "Y-comp. ang. acc.",
26 "Z-comp. ang. acc.",
27 ],
28 "lineColors": ["#ff0000", "#00ff00", (0, 0, 255), (0, 0, 0)],
29}
30
31# Create fonts and colors
32fontAxisText = Font(family="Arial", style="regular", size=14)
33legendBorderColor = Color(rgb=(204, 204, 204))
34datumColor = Color(hex="#ff0000")
35
36# Loop over pages
37for index in range(1, 3):
38 ap = ses.get(Page)
39 if index > 1:
40 ap = Page()
41 ses.setActive(Page, id=index)
42
43 # Define page attributes
44 ap.setAttributes(layout=9, title="HG Demo " + str(index))
45 i = 0
46
47 # One plot per request
48 for request in plotDict.get("yRequests"):
49 i += 1
50 aw = ses.setActive(Window, window=i)
51 aw.type = "xy"
52
53 # Add curves from dictionary
54 for component, color in zip(
55 plotDict.get("yComponents"), plotDict.get("lineColors")
56 ):
57 cu = CurveXY(
58 xFile=plotFile,
59 xType="file",
60 xDataType="Time",
61 xRequest="Time",
62 xComponent="Time",
63 yFile=plotFile,
64 yType="file",
65 yDataType="Angular Acceleration",
66 yRequest=request,
67 yComponent=component,
68 lineColor=color,
69 label="{y.HWRequest} - {y.HWComponent}",
70 )
71
72 # Add curve math SAE filter
73 cu.mathAdd("SAE", padding=1, direction=1, filterClass=15)
74
75 # Create and Modify curve math trim operation
76 mathTrim = cu.mathAdd("trim")
77 mathTrim.setAttributes(start=20, end=140)
78
79 # Add vertical datum line at curve max
80 dv = DatumVertical(
81 position="c1.x[indexofmax(c1.y)]",
82 label="Y Max",
83 labelLocation="upperCenter",
84 lineColor=datumColor,
85 )
86
87 # Edit vertical axis label using font object
88 av = ses.get(AxisVertical, curve=cu)
89 av.text = "Max of Resultant = {max(c1.y)}"
90 av.font = fontAxisText
91
92 # Modify Header values
93 header = ses.get(Header)
94 header.setAttributes(visibility=True, text="Demo Window " + str(i))
95
96 # Modify Legend Style
97
98 legend = ses.get(Legend)
99 legend.setAttributes(
100 borderColor=legendBorderColor,
101 borderStyle=3,
102 placement="insideRightBottomCorner",
103 )
104
105 # Create note at maximum of curve 3
106 note = Note(
107 attachTo="curve",
108 attachmentCurve=1,
109 attachmentPoint="indexofmax(c1.y)",
110 text="Max = {y} @ {x}",
111 autoPosition=True,
112 autoPositionStrategy="right",
113 curveColor=True,
114 )
115
116 # Update() recalculates, fits and draws
117 aw.update()
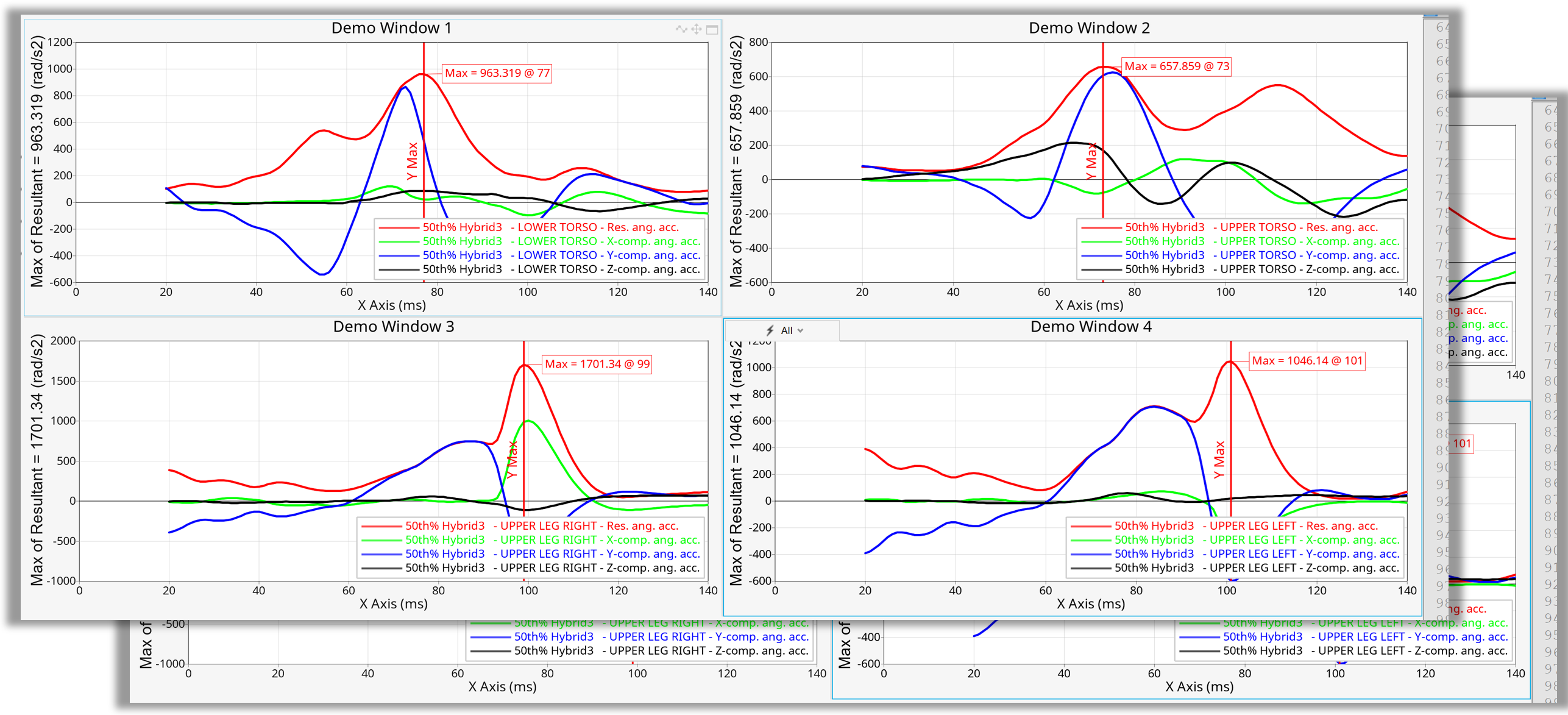
Figure 10. Output of ‘Create Multiple Plot Layout’
Example 06 - Get Channel Info#
Get channel info from plot file#
1from hw import *
2from hw.hg import *
3import os
4
5scriptDir = os.path.abspath(os.path.dirname(__file__))
6plotFile = os.path.join(scriptDir, "plot", "ANGACC")
7
8dtIndex = 1
9dataFile = DataFile(plotFile)
10dataTypeList = dataFile.getDataTypes()
11requestList = dataFile.getRequests(dataTypeList[dtIndex])
12componentList = dataFile.getComponents(dataTypeList[dtIndex])
13metaList = dataFile.getMetaDataList(dataTypeList[1], requestList[0], componentList[0])
14metaValue = dataFile.getMetaDataValue(
15 dataTypeList[1], requestList[0], componentList[0], "HWUnit"
16)
17
18print("Channel Info")
19print("File = " + plotFile)
20print("Reader Version = " + str(dataFile.readerVersion))
21print("Data Types")
22for dataType in dataTypeList:
23 print(" " + dataType)
24print("Requests of DataType = " + dataTypeList[dtIndex])
25for request in requestList:
26 print(" " + request)
27print("Components of DataType = " + dataTypeList[dtIndex])
28for component in componentList:
29 print(" " + component)
30print("Meta Data Label = " + metaList[0])
31print(" Value = " + metaValue)
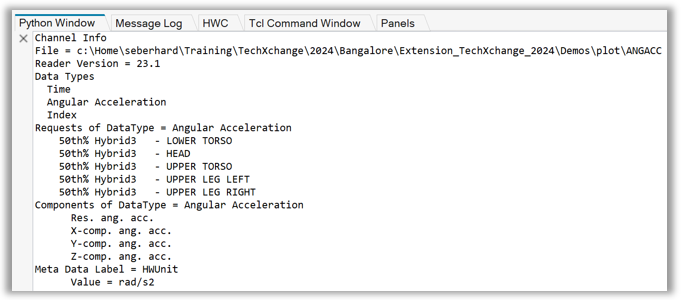
Figure 11. Console Output of ‘Get Channel Info’
Example 07 - Create Data Frames from Plot Files#
Create and plot NumPy and Pandas Data Frames#
1from hw import *
2from hw.hg import *
3import os
4import matplotlib.pyplot as plt
5
6scriptDir = os.path.abspath(os.path.dirname(__file__))
7plotFile = os.path.join(scriptDir, "plot", "ANGACC")
8
9dtIndex = 1
10dataFile = DataFile(plotFile)
11dataTypeList = dataFile.getDataTypes()
12requestList = dataFile.getRequests(dataTypeList[dtIndex])
13componentList = dataFile.getComponents(dataTypeList[dtIndex])
14
15dataFileQuery = DataFileQuery(plotFile)
16dataFileQuery.dataType = dataTypeList[dtIndex]
17dataFileQuery.requests = "all"
18dataFileQuery.components = "all"
19dataFileQuery.includeTime = True
20
21print("Numpy Array")
22numpyArr = dataFileQuery.run("numpy")
23print(numpyArr)
24
25print()
26print("Plot Pandas Data Frame")
27pandasDataFrame = dataFileQuery.run("pandas")
28pandasDataFrame.plot(figsize=(25, 20))
29plt.show()
Figure 12. Console Output of ‘Create Data Frames from Plot Files’
Example 08 - Complex Curve#
Create and edit complex curves#
1from hw import *
2from hw.hg import *
3
4scriptDir = os.path.abspath(os.path.dirname(__file__))
5plotFile = os.path.join(scriptDir, "plot", "control_arm_sol111.pch")
6
7s = Session()
8s.new()
9# evalHWC('hwd window type="HyperGraph 2D"')
10w = s.get(Window)
11w.type = "complex"
12w.axisMode = "ri"
13
14yrCompList = ["REL | X-Trans", "REL | Y-Trans", "REL | Z-Trans"]
15yiCompList = ["IMG | X-Trans", "IMG | Y-Trans", "IMG | Z-Trans"]
16colorList = ["#ff0000", "#00ff00", "#0000ff"]
17
18for yrComp, yiComp, color in zip(yrCompList, yiCompList, colorList):
19 cu = CurveComplex(
20 xFile=plotFile,
21 label=yrComp.split(" ")[-1],
22 xSource="file",
23 xDataType="Frequency [Hz]",
24 xRequest="Frequency [Hz",
25 xComponent="Frequency [Hz",
26 yrFile=plotFile,
27 yrSource="file",
28 yrDataType="Displacements",
29 yrRequest="Point id 2086",
30 yrComponent=yrComp,
31 yiFile=plotFile,
32 yiSource="file",
33 yiDataType="Displacements",
34 yiRequest="Point id 2086",
35 yiComponent=yiComp,
36 lineColor=color,
37 window=1,
38 page=1,
39 )
40
41cu.shadeArea = True
42cu.shadeStyle = "betweenCurves"
43cu.shadeSecondCurve = 2
44cu.shadeColor = (0, 0, 0)
45cu.shadeAlpha = 0.2
Figure 13. Complex Curve
Example 09 - Plotting Curves from Single File#
Advanced Build Plots functionality via Python#
1from hw import *
2from hw.hg import *
3import os
4
5scriptDir = os.path.abspath(os.path.dirname(__file__))
6plotFile = os.path.join(scriptDir, "plot", "ANGACC")
7
8ses = Session()
9ses.new()
10# evalHWC('hwd window type="HyperGraph 2D"')
11win = ses.get(Window)
12win.type = "xy"
13
14dataTypeList = ["Time", "Angular Acceleration", "Index"]
15dataType = dataTypeList[1]
16
17createCurves = CreateCurvesByFile()
18createCurves.file = plotFile
19createCurves.yDataType = dataType
20createCurves.yRequest = "all"
21createCurves.yComponent = "all"
22createCurves.layoutType = 1
23createCurves.layoutOrganisation = "onePlotPerRequest"
24createCurves.run()
Figure 14. Plotting Curves from Single File
Example 10 - Plotting Curves from Multiple Files#
Advanced Build Plots functionality for plotting from multiple files#
1from hw import *
2from hw.hg import *
3import os
4
5scriptDir = os.path.abspath(os.path.dirname(__file__))
6multipleFileDir = os.path.join(scriptDir, "doe_1")
7plotFile = os.path.join(scriptDir, "doe_1", "run__00001", "m_1", "boxbeamT01")
8
9ses = Session()
10ses.new()
11win = ses.get(Window)
12win.type = "xy"
13
14dtIndex = 1
15dataFile = DataFile(plotFile)
16dataTypeList = dataFile.getDataTypes()
17requestList = dataFile.getRequests(dataTypeList[dtIndex])
18componentList = dataFile.getComponents(dataTypeList[dtIndex])
19
20multipleFiles = MultipleFiles()
21multipleFiles.name = "FS1"
22multipleFiles.directory = "c:/Home/seberhard/Projects/Python/models/Plot/doe_1"
23multipleFiles.fileList = [
24 "run__00001/m_1/boxbeamT01",
25 "run__00002/m_1/boxbeamT01",
26 "run__00003/m_1/boxbeamT01",
27 "run__00004/m_1/boxbeamT01",
28]
29multipleFiles.labelPrefixSuffix = "dir2"
30multipleFiles.typePrefixSuffix = "prefix"
31fileSet = multipleFiles.createFileSet()
32
33createCurves = CreateCurvesByFile()
34
35createCurves.file = multipleFiles
36
37createCurves.yDataType = dataTypeList[1]
38createCurves.yRequest = requestList[0:2]
39createCurves.yComponent = componentList
40createCurves.layoutType = 1
41createCurves.layoutOrganisation = "onePlotPerRequest"
42createCurves.run()
Figure 15. Plotting Curves from Multiple Files