GUI Toolkit Examples#
Example 01 - Custom Dialog#
Custom Dialog#
1from hw import *
2from hw.hv import *
3from hwx.xmlui import gui
4from hwx import gui as gui2
5import os
6
7
8def MyCustomGui():
9
10 # Set of functions used as commands linked to various widgets
11 def onClose(event):
12 dialog.Hide()
13
14 def onRun(event):
15 dialog.Hide()
16 gui2.tellUser("Done!")
17
18 def onModified():
19 # Function to update the output label with the slider value when
20 # the slider is interactively used
21 output.value = str(f"Output Quality: {slider.value}")
22
23 def comboboxFunc(event):
24 # output.text = event.value
25 pass
26
27 # Creating objects for various UI widgets
28 label1 = gui.Label(text="Model File")
29 modelFile = gui.OpenFileEntry(placeholdertext="Model File")
30 label2 = gui.Label(text="Result File")
31 resultFile = gui.OpenFileEntry(placeholdertext="Result File")
32 label3 = gui.Label(text="Screenshot Folder")
33 folderSel = gui.ChooseDirEntry(tooltip="Select output directory")
34 slider = gui2.Slider(maxvalue=100, tracking=False, value=10, command=onModified)
35 output = gui2.Label(f"Output Quality: {slider.value}")
36 formats = (("jpeg", "JPEG"), ("png", "PNG"))
37 buttons = gui2.HRadioButtons(formats)
38 levels = (("lvl1", "Level 1"), ("lvl2", "Level 2"), ("lvl3", "Level 3"))
39 combobox = gui2.ComboBox(levels, command=comboboxFunc)
40
41 # Defining the pair of custom buttons at the bottom of the dialog
42 close = gui.Button("Close", command=onClose)
43 create = gui.Button("Run", command=onRun)
44
45 # Creating a vertical frame to organize all the widgets.
46 # We are using integer values or <-> spacer to control the spacing between widgets.
47 mainFrame = gui.VFrame(
48 (label1, 10, modelFile),
49 (label2, 10, resultFile),
50 (label3, 10, folderSel),
51 (output, 10, slider),
52 (buttons, "<->"),
53 (combobox, "<->"),
54 (create, close),
55 )
56
57 # Creating a dialog instance that hosts and displays all the widgets constructed above
58 dialog = gui.Dialog(caption="Example Dialog")
59 dialog.recess().add(mainFrame)
60
61 # The xmlui.gui Dialog uses two bottom buttons by default. We are hiding them as we created
62 # our own Run and Close buttons to demonstrate what could be controlled.
63 dialog.setButtonVisibile("ok", False)
64 dialog.setButtonVisibile("cancel", False)
65
66 # Displaying the dialog with prescribed dimensions
67 dialog.show(width=400, height=100)
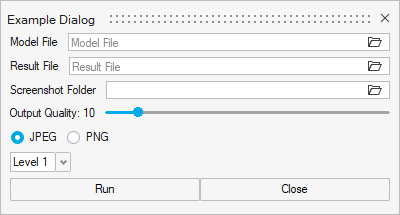
Figure 1. Custom dialog containing various widgets